What are you required to be able to complete in order to develop an application capable of recognizing living objects using React? (r) (r)
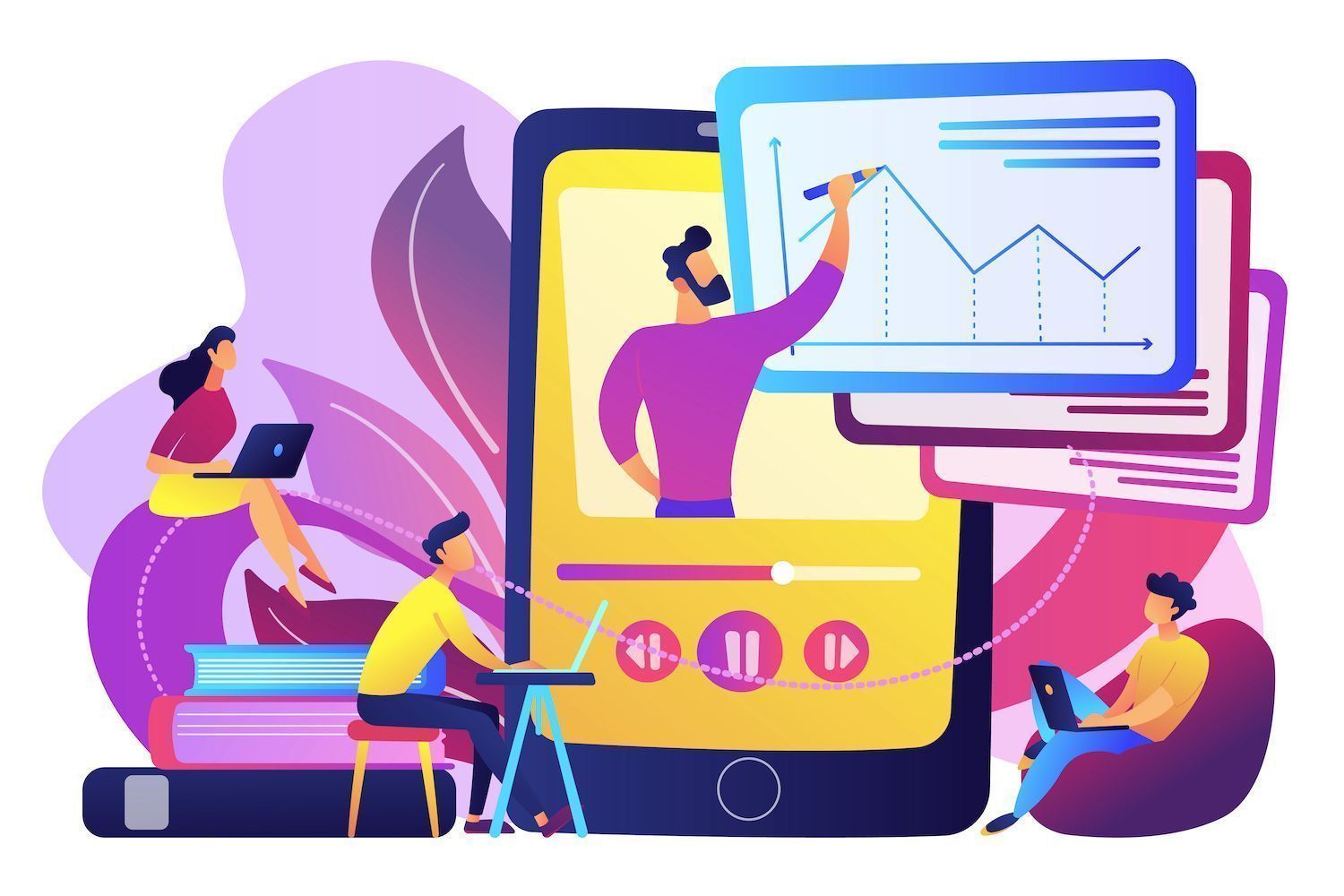
-sidebar-toc>
Cameras are getting better and becoming more advanced. The ability to identify objects at a moment's notice is rapidly becoming a popular alternative. From autonomous vehicles to modern surveillance equipment to AR applications, AR technology is used to various purposes.
Computer Vision is the nebulous word employed to define a process that makes use of computers and cameras to accomplish various tasks. This is, as mentioned previously it's a vast and complex area. Most people aren't convinced that they're able to start participating in the immediate looking for things using a Google search engine.
The scene
This is a comprehensive review of the most important techniques that are used in this article:
- TensorFlow.js: TensorFlow.js is a JavaScript library that brings the benefits of machine learning into your browser. It allows you to download models that are trained to complete the task of object recognition to provide them to the web browser. It eliminates the need to do complex server-side processing.
- Coco SSD It's an application for object recognition that is taught. It's also known by the title of Coco SSD that is a lightweight model that is capable to identify the vast majority of objects that are commonly utilized. While Coco SSD is a powerful tool, you need to recognize its reality that it was designed using a variety of objects. If you're searching for an exact characteristic that you are able to identify and then create an individual model using TensorFlow.js with this guide.
Design a completely fresh React project
- Make a brand fresh React project. It is simple to do with these simple steps:
NPM create vite@latest -object detection React template
This will be the very first React project that you can develop using..
- Following that, you'll capable of downloading TensorFlow as well as Coco SSD. Coco SSD libraries with these commands inside the project:
npm i @tensorflow-models/coco-ssd @tensorflow/tfjs
It's time to create your application.
Configuring the application
Before writing the code to create the logic necessary to recognize objects, look at the code that was written in this instruction. The user interface in the application might include:
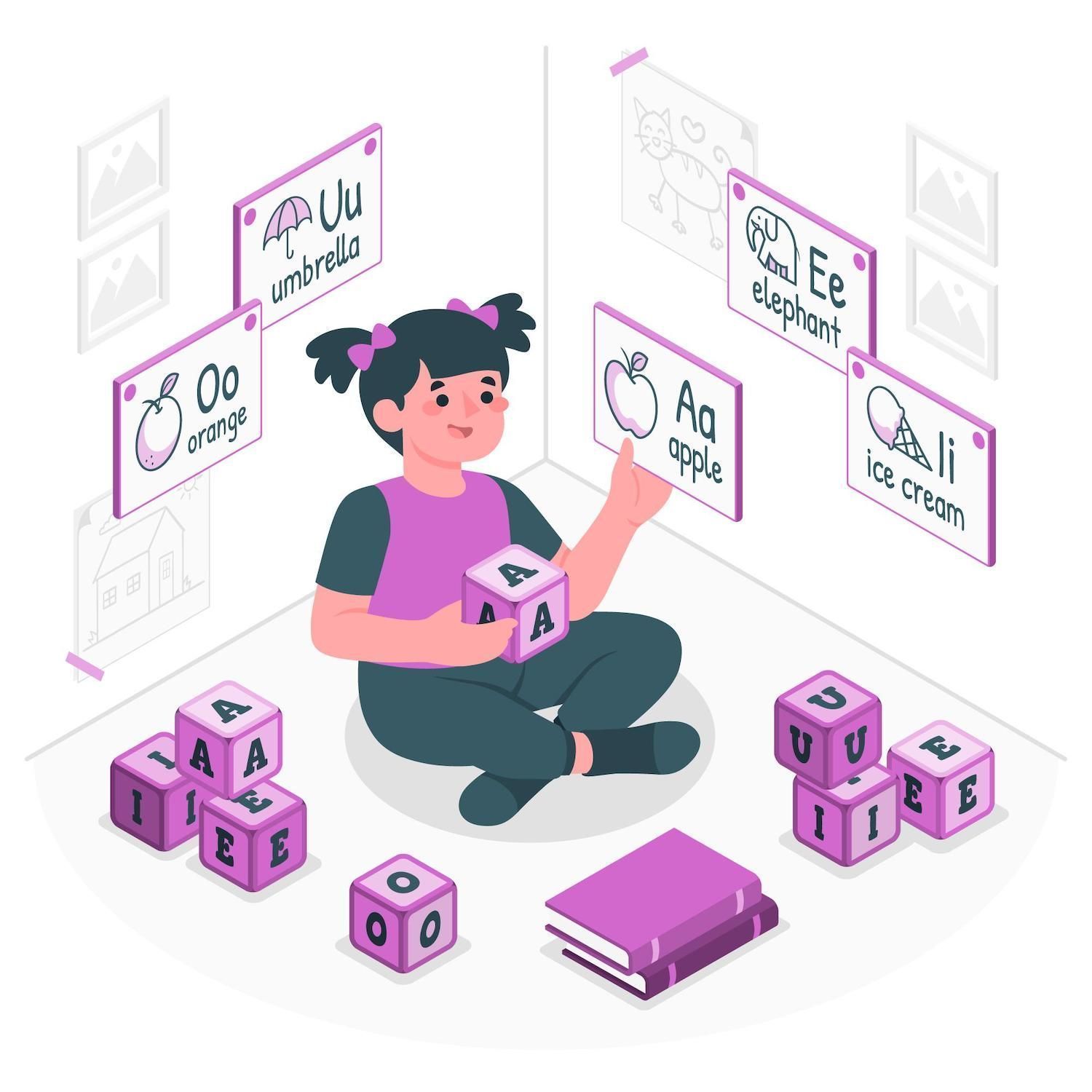
When users press the Start Webcam button upon clicking on the Start Webcam button, they're required to authorize the application to make use of webcam feeds. When permission is granted then the application will show the live stream of the webcam. It is also able to identify any things it observes within the stream. It forms an equilateral triangular shape in order to illustrate what it is seeing inside the feed. Then, it is able to label those items. It is then able identify the items.
The first step you have to accomplish is creating an intuitive user interface to the application. Copy these steps to app.jsx. App.jsx file:
import ObjectDetection from './ObjectDetection'function App() return ( Image Object Detection ); Export default App
The code fragment functions as the page's header. It also includes the custom component "ObjectDetection"
. It takes the feed from a camera and determine objects on the spur at the right time.
To build this component make an entirely new file with the name ObjectDetection.jsx in your homedirectory then paste the following code into it:
UseEffect useState and'react'. Const objectDetection = () Const videoRef = useRef(null) Const [isWebcamStartedsetIsWebcamStarted], useState(false) Const setWebcam to be in sync () = /StopWebcam () > // TODO; return ( isWebcamStarted? "Stop" : "Start" Webcam isWebcamStarted ? : ); ; export default ObjectDetection;
You can implement the code for building the startWebcam. "startWebcam"
function:
const startWebcam = async () => try setIsWebcamStarted(true) const stream = await navigator.mediaDevices.getUserMedia( video: true ); if (videoRef.current) videoRef.current.srcObject = stream; catch (error) setIsWebcamStarted(false) console.error('Error accessing webcam:', error); ;
The system will request users to authorize access to the webcam. Once granted, the system modifies the video stream of the webcam. video
will show the live stream of the webcam for anyone who is connected to the webcam.
If the app is not capable of connecting to the feeds of the camera (possibly because of the absence of a webcam in the device or a different reason for why the user wasn't granted access) it will show an error message on the console. The console may display an error message which will explain the source of this issue to the user.
The next step is replacing stopWebcam using stopWebcam. stopWebcam
function with this code:
const stopWebcam = () => const video = videoRef.current; if (video) const stream = video.srcObject; const tracks = stream.getTracks(); tracks.forEach((track) => track.stop(); ); video.srcObject = null; setPredictions([]) setIsWebcamStarted(false) ;
The code scans for videos which can be accessed via webcam objects. webcam
object. This will then stop each of them. Following that it changes to change its webcam's
status to the real number.
.
Similar to the scenario. It is possible to launch the application and check whether it's able to connect to and display the feed of the webcam.
The code must be placed into the index.css file to ensure that the app looks exactly like the one you had earlier seen.
#root font-family: Inter, system-ui, Avenir, Helvetica, Arial, sans-serif; line-height: 1.5; font-weight: 400; color-scheme: light dark; color: rgba(255, 255, 255, 0.87); background-color: #242424; min-width: 100vw; min-height: 100vh; font-synthesis: none; text-rendering: optimizeLegibility; -webkit-font-smoothing: antialiased; -moz-osx-font-smoothing: grayscale; a font-weight: 500; color: #646cff; text-decoration: inherit; a:hover color: #535bf2; body margin: 0; display: flex; place-items: center; min-width: 100vw; min-height: 100vh; h1 font-size: 3.2em; line-height: 1.1; button border-radius: 8px; border: 1px solid transparent; padding: 0.6em 1.2em; font-size: 1em; font-weight: 500; font-family: inherit; background-color: #1a1a1a; cursor: pointer; transition: border-color 0.25s; button:hover border-color: #646cff; button:focus, button:focus-visible outline: 4px auto -webkit-focus-ring-color; @media (prefers-color-scheme: light) :root color: #213547; background-color: #ffffff; a:hover color: #747bff; button background-color: #f9f9f9; .app width: 100%; display: flex; justify-content: center; align-items: center; flex-direction: column; .object-detection width: 100%; display: flex; flex-direction: column; align-items: center; justify-content: center; .buttons width: 100%; display: flex; justify-content: center; align-items: center; flex-direction: row; button margin: 2px; div margin: 4px;
The app.css file must be removed. App.css file to make sure you do not damage your components' appearance. Now you are ready to incorporate the technology needed for real-time object recognition to your app.
Implement real-time detection for objects
- First steps is to import the data into Tensorflow along with Coco SSD in the middle of ObjectDetection.jsx :
import * as cocoSsd from '@tensorflow-models/coco-ssd'; import '@tensorflow/tfjs';
- Add a new condition to the
ObjectDetection
component, so as to maintain the prediction array generated by Coco SSD model. Coco SSD model: Coco SSD model:
const [predictions setPredictions, useStatesetPredictions, useState ([]);
- After that, you are capable of creating an application that is loaded to your Coco SSD model. Coco SSD model. It is loaded onto Coco SSD model, loads onto Coco SSD model, collects footage and predicts:
const predictObject = async () => const model = await cocoSsd.load(); model.detect(videoRef.current).then((predictions) => setPredictions(predictions); ) .catch(err => console.error(err) ); ;
The program makes use of footage taken from the video feed to create forecasts of things that are in the feed. It offers users a variety of objects which are expected to be seen. Each one has a label which includes a percentage the confidence level, and numbers that indicate the position of the object in the video's frame.
It is essential that you turn this feature on to run videos according to the order in which frames get added. The forecasts will be later utilized and stored in this forecasts
condition. The forecasts will show boxes with labels for every identified object within the live video stream. live-stream view.
- Then you'll be able to position to utilize
this settingInterval
function to activate this feature at an recurring period. Also, you must be sure the function not active in the event that the user is shut off updates from their webcam. To avoid this, you must use theClearInterval
feature in JavaScript.Add your container for state as well as hooks for useEffect to theaffect
hooks in theelement, the detector of the objects
element, to construct anpredict
function. The program is running continuously when the webcam is active However, it will be removed from the camera once it's turned off.
const [detectionInterval, setDetectionInterval] = useState() useEffect(() => if (isWebcamStarted) setDetectionInterval(setInterval(predictObject, 500)) else if (detectionInterval) clearInterval(detectionInterval) setDetectionInterval(null) , [isWebcamStarted])
The application is designed to detect items in the image captured by the camera each 500 milliseconds. It is possible to alter the amount of milliseconds every second depending on the speed you'd like the speed of object detection however, it's important to consider the potential of using frequently. It could lead to that the program consuming large amounts of memory within the browser.
- When you've got the data for your forecast, you can now use your data to make estimates. If you've estimated some aspects of your forecast, these could be used to mark containers. Forecasts can be utilized to show your label inside the containers as well as to show the label in the live feed within the movie. In order to do this, it's necessary to change your
returning
declaration for yourlabels detection.
Enter the following details:
Return ( Is WebcamStarted ? "Stop" : "Start" Webcam isWebcamStarted ? : /* Add the tags below to show a label using the p element and a box using the div element */ predictions.length > 0 && ( predictions.map(prediction => return prediction.class + ' - with ' + Math.round(parseFloat(prediction.score) * 100) + '% confidence. ' > ) ) /* Add the tags below to show a list of predictions to user */ predictions.length > 0 && ( Predictions: predictions.map((prediction, index) => ( `$prediction.class ($(prediction.score * 100).toFixed(2)%)` )) ) );
The program displays a list of forecasts beneath the feed from the webcam. The program creates an empty area around the object which will be forecasted using coordinates from Coco SSD as well as names in the bottom of every box.
- for styling the labels and boxes correctly to style boxes and labels correctly, add this code within your index.css Index.css index.css file. index.css file:
.feed position: relative; p position: absolute; padding: 5px; background-color: rgba(255, 111, 0, 0.85); color: #FFF; border: 1px dashed rgba(255, 255, 255, 0.7); z-index: 2; font-size: 12px; margin: 0; .marker background: rgba(0, 255, 0, 0.25); border: 1px dashed #fff; z-index: 1; position: absolute;
The application is complete. It is complete. The program has been in a position to operate the server was designed to test the functionality of the program. What happens after it has been finished
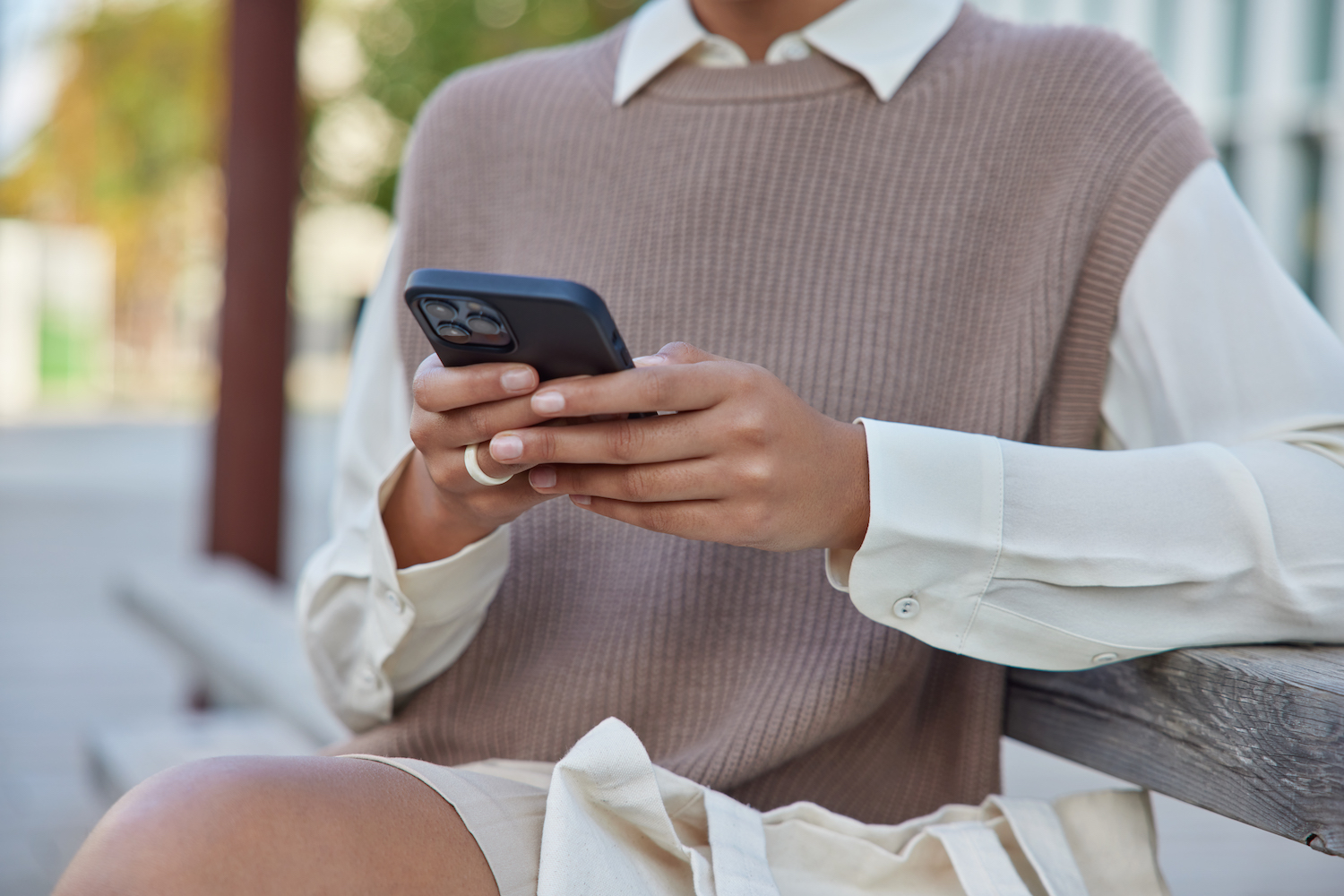
The entire code can be found on the repository GitHub. GitHub repository.
Install the app
If your repository on Git is working and functional, You can follow these steps to install Git :
- Register or create an account so that you can login to Your Dashboard. My dashboard.
- You must authorize your Git service providers.
- Select the static sites on the sidebar to left. Choose Add Site. Select to add the website.
- Select the branch you wish to join and then the repository you want to have access to.
- Assign a unique name to your site.
- Set up the building's settings according to the following model:
- Command to build:
yarn build
orNPM build
- Node version:
20.2.0
- Publish directory:
dist
- Then, click Create site.
If the app is created after which the app has been released, it is possible to choose "Visit the website" from the dashboard to launch the application. The application can be tested using multiple cameras across different platforms to determine the functionality of the app.
Summary
It's had an enormous success with creating the machine-learning technology for object recognition that operates in real-time in addition to live-time software that was created with React, TensorFlow.js, and . It lets you discover the potential of computer vision. You can also create interactive experiences through your web browser.
Take note you are using this model. Coco SSD model we used as a base. If you'd like to continue investigating the many alternatives, it is worth exploring the possibility of being in a position to alter the process of identifying objects with TensorFlow.js which lets you change the way that the software recognizes those objects which best satisfy the particular requirements of the company you work for.
There's no limit on the possibilities that you can create! The app can be the base for creating innovative applications such as Augmented Reality Experiences and also cutting-edge surveillance instruments. If you're able, in the event of launching your app via the safe platform, you are capable of making your application accessible to everyone around the world and witness the potentialities of computer vision emerge into the spotlight.
What's the most difficult issue that you've had to face and think that real-time detection of objects would be able to help solve? Please share your story in the comments section in the comment section below!
Kumar Harsh
Kumar is the creator of technical software. He manages his own home in India. He's an expert in JavaScript as well as DevOps. Get more information about the subject on his website.
The original article was published this website.
The article was posted on this site.
The article was posted by the author on the blog.
The post was published on this site.
Article was first seen on here
Article was posted on here