Learning to master Laravel Routes
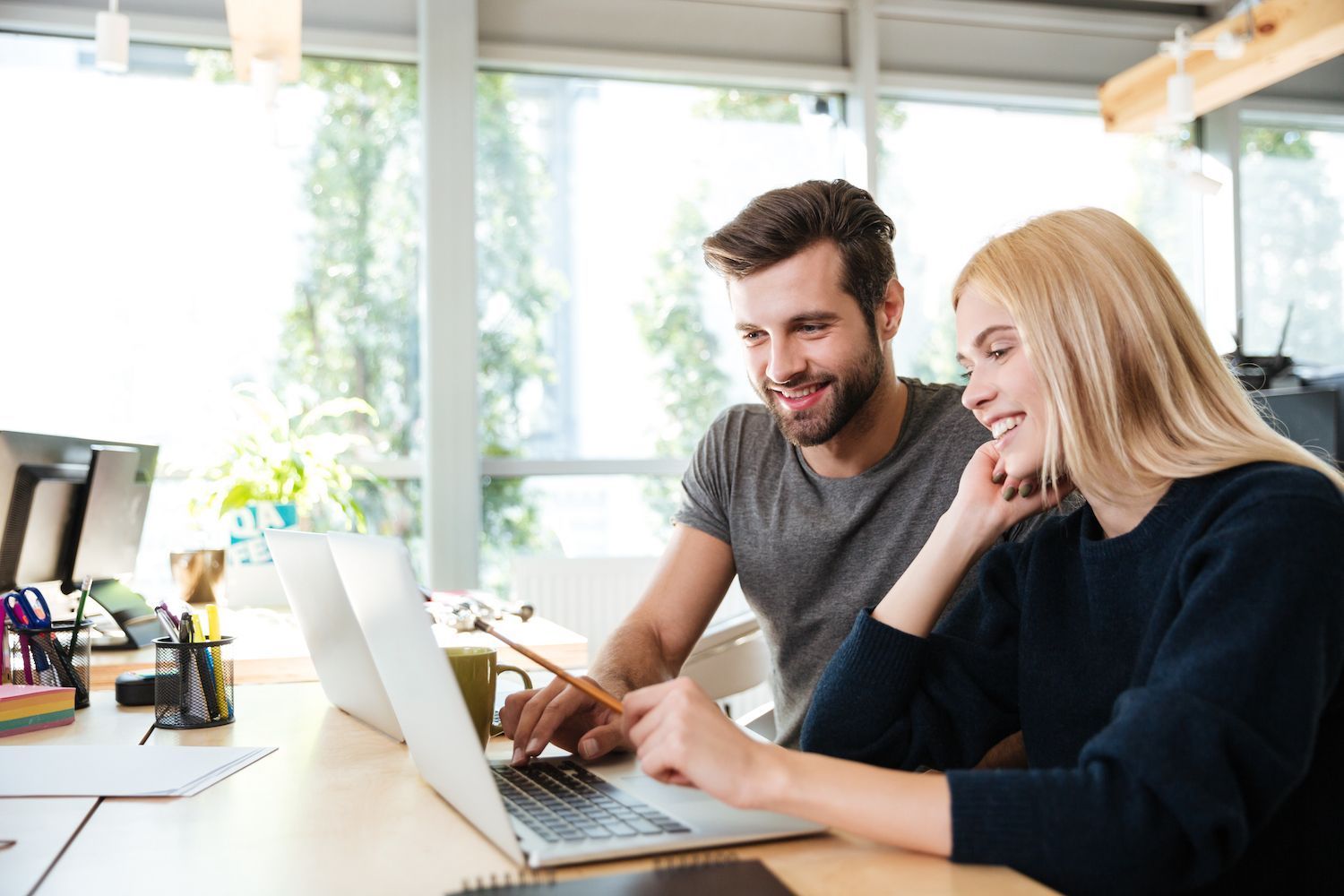
In the backend, eventually we encounter routes. The routing list is at the heart of the backend because every request the server receives is passed to the controller via the list of routes. It is dependent on the controller or actions.
Backend Routing and Cross-Site Scripting in Laravel
On servers, you can find two routes: public and private. The public routes may provide the possibility of XSS.
The issue arises due to the fact that a user may be taken by a website that does not require an account token. They could instead , go to one that has one and get access using no token.
The ideal solution to the issue is to make use of an entirely brand new HTTP header, and include "referrer" in the URL to avoid this situation.
'main' => [ 'path' => '/main', 'referrer' => 'required,refresh-empty', 'target' => Controller\DashboardController::class . '::mainAction' ]
Basic Routing for Laravel
The way that routes are built in Laravel these routes are constructed inside the web.php and api.php files, and Laravel has two routes available by default. One of them is designed for WEB as well as one that is intended for API. API.
These routes reside in the routes/ folder, but they are loaded in the Providers/RouteServiceProvider.php
Instead of doing this we can load the routes directly inside the RouteServiceProvider.php skipping the routes/ folder altogether.
Redirects
// Simple redirect Route::redirect("/class", "/myClass"); // Redirect with custom status Route::redirect("/home", "/office", 305); // Route redirect with 301 status code Route::permanentRedirect("/home", "office");
For the redirect route, it is not permitted to make use of the "destination" or "status" keyword in order to establish parameters as they are protected by Laravel.
// Illegal to use Route::redirect("/home", "/office/status");
Views
The Views files The Views files . blade.php files that are used as the frontend of the Laravel application. Blade is the templating engine. It's the most commonly used method to build a full stack app using Laravel.
If the goal of our routing is to show a view it is possible to make use of the view method within the Route facade. It is able to take a parameter from a route as well as the name of the view along with an array of value to be passed to the view.
// When the user will enter the 'my-domain.com/homepage // the homepage.blade.php file will be rendered Route::view("/homepage", "homepage"); // Let's assume our view wants to say "Hello, name", // by passing an optional array with that parameter // we can do just that and if the parameter is missing but // it is required in the view, the request will fail and throw an error Route::view('/homepage', 'homepage', ['name' => ""]);
Route Liste
When an application grows in its size, so will the number of requests to be handled. In addition, with the huge volume of data , comes plenty of confusion.
That's where the art route:list can help. It provides a list of the various routes that are defined by the application as well as their middlewares and controllers.
php artisan route:list
The program will provide an overview of all possibilities of routes without middleware. To accomplish this, we need to utilize the flag with the "-v"
php artisan route:list -v
If you might be employing Domain Driven Design and your routes could have names that are unique to them it is possible to use the filters offered through this choice.
php artisan route:list -path=api/account
This only shows the routes beginning by the API/Account. Account/API..
However, you can tell Laravel to either not add or exempt the third-party defined routes using options such as that of -except-vendor or only-vendor options.
Route Parameters
In some cases, we'll need to record certain elements of the URI via the route like an ID number for the user or token. It is possible to do this through the creation of parameters for the route, which is always encased inside the ' ' braces and must contain only characters.
If our routes contain dependencies within the callbacks The Laravel Service Container will automatically include them.
use Illuminate\Http\Request; use Controllers/DashboardController; Route::post('/dashboard/id, function (Request $request, string $id) return 'User:' . $id; Route::get('/dashboard/id, DashboardController.php);
Mandatory Parameters
The criteria that must be met to be fulfilled by the user are the ones we're not allowed to circumvent when making attempts to call. If we attempt, a error can be reported.
Route::post("/gdpr/userId", GetGdprDataController.php");
Now inside the GetGdprDataController.php we will have direct access to the $userId parameter.
The public function __invoke(int userId) // Make use of the username we received...
The route may include many parameters, and these may be included in callbacks to controllers or routes in accordance with the order they are entered:
Do you want to know the steps we took to boost the number of visitors to our website by 1,000 per percent?
Join over 20,000 subscribers for our weekly newsletter, which includes insider WordPress tricks!
// api.php Route::post('/gdpr/userId/userName/userAge', GetGdprDataController.php); // GetGdprDataController.php public function __invoke(int $userId, string $userName, int $userAge) // Use the parameters...
Optional Parameters
In the event that you wish to carry out the action in a specific technique only when the parameter is available and doesn't affect the application in general or even the entire application you can create another parameter.
It is evident by the letters '?' added to them.
Route::get('/user>' (function (int age = null) *if (!$age) Log::info("User isn't an age that is") Otherwise Log::info("User's age is " . $age); Route::get('/user/"; method (int"$name" is "John Doe") Log::info("John Doe")Log::info("User's name begins with " . $name);
Route Wildcard
"Where Method "where method" will take the names of the parameters, along with the regex rules utilized to validate the parameters. The default is to accept the first parameter, but when we're faced with many options one can give an array that contains the names of every parameter in keys as well as the value of the rule. Laravel can sort them out to us.
Route::get('/user/age', function (int $age) // ->where('age', '[0-9]+'); Route::get('/user/age', function (int $age) // ->where('[0-9]+'); Route::get('/user/age/name', function (int $age, string $name) // ->where(['age' => '[0-9]+', 'name' => '[a-z][A-z]+');
It is possible to take this one step further by applying it to every route in our application with the use of the pattern technique to the Route facade
Route::pattern('id', '[0-9]+');
It causes every ID parameter to be validated through this regex phrase. Once we've created the expression, it'll be instantly applied to any potential routes using that name.
Route::get('/find/query', function ($query) // )->where('query', , '. *');
Named Routes
How can we best to create name-brand Routes
An easy way to accomplish this is through the name method, which can be linked to the Route facade. Names should be unique.
Route: get ('/', function () )->name("homepage");
Route Groups
Route groups allow you to connect middleware as well as other characteristics across a range of routes without the need to create it for each and every route.
Middleware
To assign a middleware one of these techniques, it is necessary to include the middlewares within the group prior to using the group method. A different aspect to consider is the fact that middlewares will be performed exactly the same way as they're included in the group.
Route:middleware(['AuthMiddleware', 'SessionMiddleware'])->group(function () Route::get('/', function() ); Route::post('/upload-picture', function () ); );
Controllers
If a group has the same controller, it's possible to utilize the controller method to identify the controller which is shared with all the routes of the group. Now we have to specify the method that each route will utilize to contact.
Route::controller(UserController::class)->group(function () Route::get('/orders/userId', 'getOrders'); Route::post('/order/id', 'postOrder'); );
Subdomain Routing
The routes could be applied to subdomain-specific routing. The domain can be picked up and a portion of the subdomain to be used for our controller and routes. By using the domain method of the Route facade, it is possible to find the routes are used for a domain
Route::domain('store.enterprise.com')->group(function() Route::get('order/id', function (Account $account, string $id) // Your Code );
Name Prefixes as well Prefixes
If you are confronted with a set of routes, instead of changing between them, it's possible to utilize the tools that Laravel include, for instance, the name and the prefix appearing on the Route facade.
Methods of prefixing could be used to prefix all routes within the group by the given URI as well as making use of the name method which can be employed to prefix each route's name with a certain amount of characters.
It allows us to design similar routes to Admin without the need to modify each name and prefix to separate them.
Route::name('admin. ")->group(function() Route::prefix("admin")->group(function() Route::get('/get')->name('get'); Route::put('/put')->name(put'); Route::post('/post')->name('post'); ); );
In the near future, the URI for these routes is likely to contain admin/get as well as admin/put. along with the names admin.get, admin.put, admin.post.
Route Caching
What is the meaning of Route Caching?
The caching of routes will cut down on the time needed for each application to track its routes.
Running php artisan route:cache an instance of Illuminate/Routing/RouteCollection is generated and after being encoded, the serialized output is written to bootstrap/cache.routes.php.
It's essential to make use of Route Caching
By not using the route cache feature which Laravel allows, we're slowing our website quicker than what is needed and also reducing users' retention as well as the general pleasure of our site.
Based on the scope of your application as well as the quantity of possible routes. In the case of the use of just 1 command could speed up your application by 1.3x times, or even 5x times.
Summary
Routing is the core to Backend development. Laravel excels at this through its intuitive method of organizing and delineating routes.
- Simple setup and administration in My dashboard. My dashboard
- Help is always on hand.
- The most effective Google Cloud Platform hardware and network, that is driven by Kubernetes to ensure maximum capacity
- Enterprise-level Cloudflare integration that improves performance and security.
- A global audience can be attained through as many as 35 data centers, as in addition to more than 275 PoPs in every corner of all over.
The post was published on this website.
Article was first seen on here