Laravel API Test and create the API for Laravel -(r) (r)
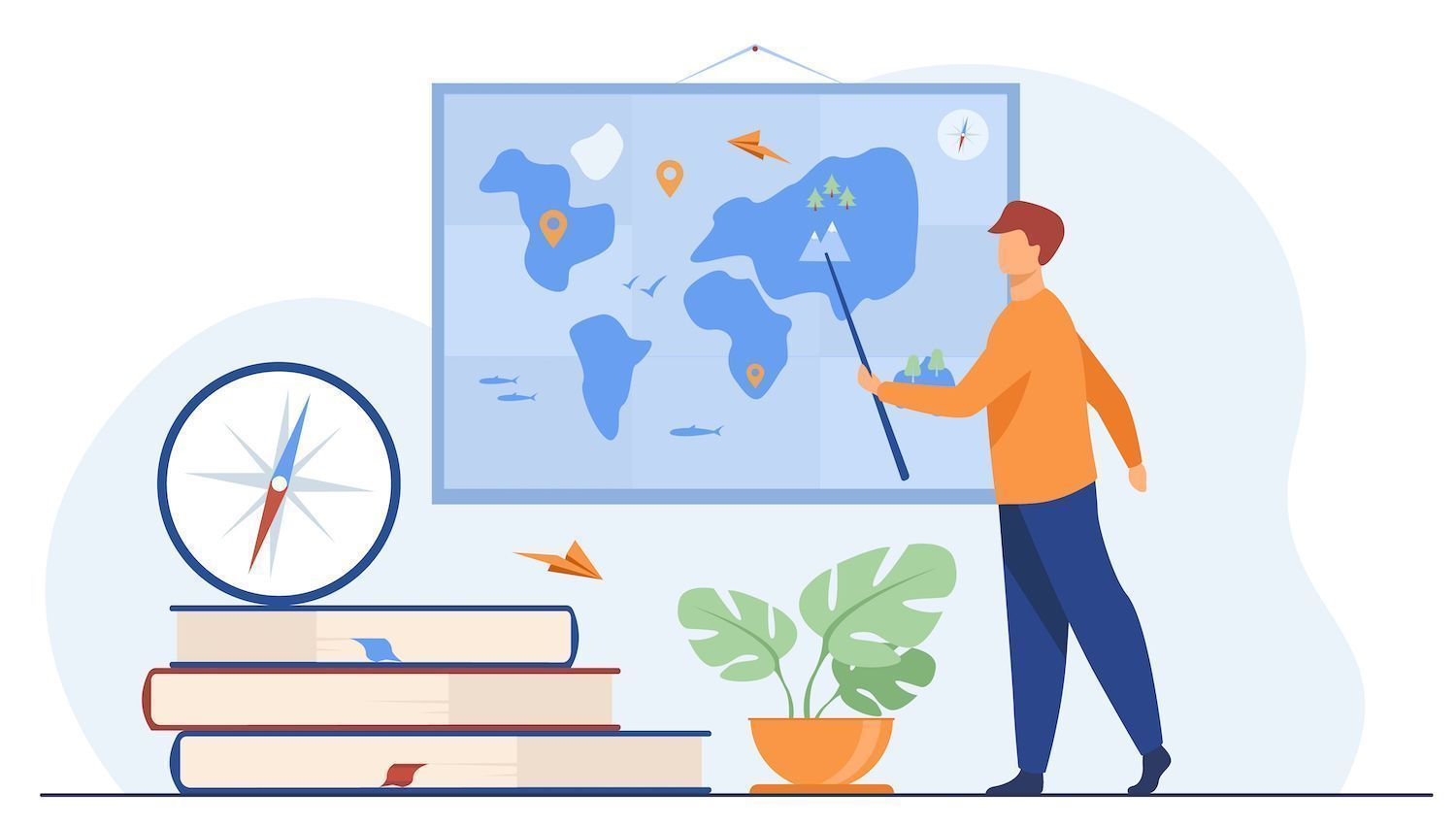
Please send the details to
Laravel Eloquent provides a straightforward method for connecting to your database. It's an object relational mapping (ORM) which simplifies the complicated database procedure by establishing a framework which lets you interact with tables.
Furthermore, Laravel Eloquent has excellent tools to create and test APIs. The tools are able to assist the developers to develop APIs. In this article you'll discover how easy to create APIs and then test them in Laravel.
The circumstances
For you to get started on how to proceed, here's all the details you'll require
- Version 8.8 of HTML0 is being released accessible for download using Laravel as well as version 9.
- Composer
- Postman
- XAMPP
API Basics
Design a new Laravel project in conjunction with an experienced composer
:
composer create-project laravel/laravel laravel-api-create-test
In order to start the server, you must run this command. Server is launched at port 800:
cd laravel-api-create-test php artisan serve
It is strongly suggested that you review the following screen:
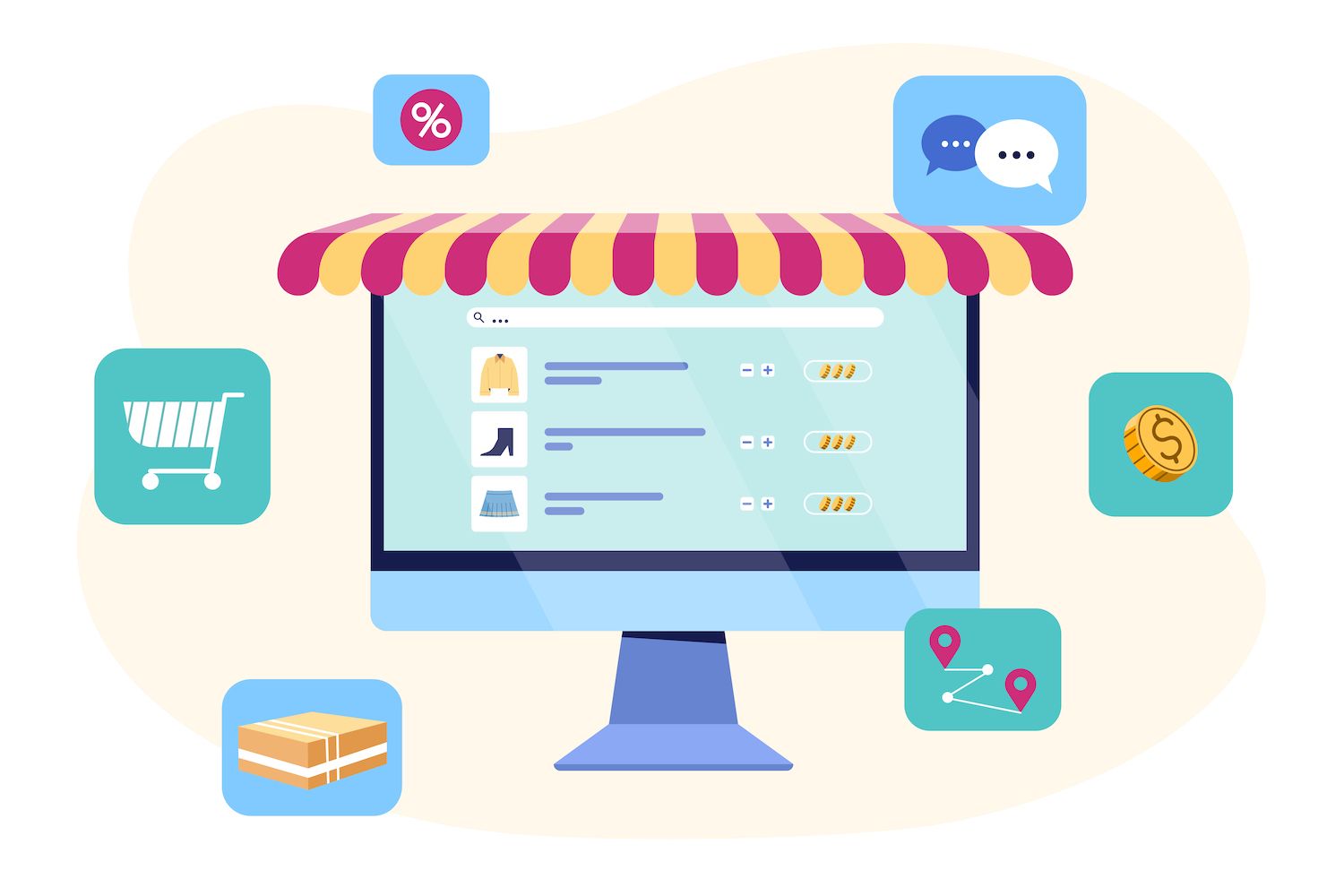
Models made with"-m" in their "-m
flag to permit the migration to be performed applying the code classified according to:
php artisan make:model Product -m
After that, you can upgrade the file with the needed field. Add title and description fields for the product model and these two table fields inside the database/migrations/date_stamp_create_products_table.php file.
$table->string('title'); $table->longText('description');
The next step is making those fields filled up to capacity. Inside app/Models/Product.php, make title
and description
fillable fields.
protected $fillable = ['title', 'description'];
What are the most important things you need to know about the Controller?
Create a controller that can operate the device with the command. This will create the app/Http/Controllers/Api/ProductController.php file.
php artisan make:controller Api\\ProductController --model=Product
Design the logic that will make and retrieve objects. In an index
method, you can integrate this code into your system to a examine each object.
products = $products x all :(); Get the results ()->json(["status" is"false" as well "products" exceeds"$products");
In the next step, you must add the StoreProductRequest
class that will store the new items inside the database. This class will be placed over the existing file.
public function store(StoreProductRequest $request) $product = Product::create($request->all()); return response()->json([ 'status' => true, 'message' => "Product Created successfully! ", 'product' => $product ], 200);
This is the next step to initiate by making a request. This is accomplished by using the following command:
php artisan make:request StoreProductRequest
If you want to add validations, you can use the app/Http/Requests/StoreProductRequest.php file. It's a test that doesn't include validation.
What Are You Required To Create A Route
The final thing to do before making your try using the API is to develop a completely new API. This is why you should include this code in the routes/api.php file. The file must contain the usage
declaration at the top of the file. Additionally, you should include the routing
assertion within the body of the file.
use App\Http\Controllers\Api\ProductController; Route::apiResource('products', ProductController::class);
Before you start testing the API, make sure that a product table in the database. If it is not there you can create it using the help by a control panel for instance XAMPP. In addition, you can employ these commands to change the table.
php artisan migrate
Which is the best way to try an API? API
Before testing the API, ensure that the authorize
method inside the app/Http/Requests/StoreProductRequest.php is set to return true
.
Create a new product by using Postman. Start by hitting a POST
request to this URL: http://127.0.0.1:8000/api/products/. Because this is a POST request for an
application that will facilitate the development of a brand new product, it is essential to include a JSON object with the description as well as a name.
"title":"Apple", "description":"Best Apples of the world"
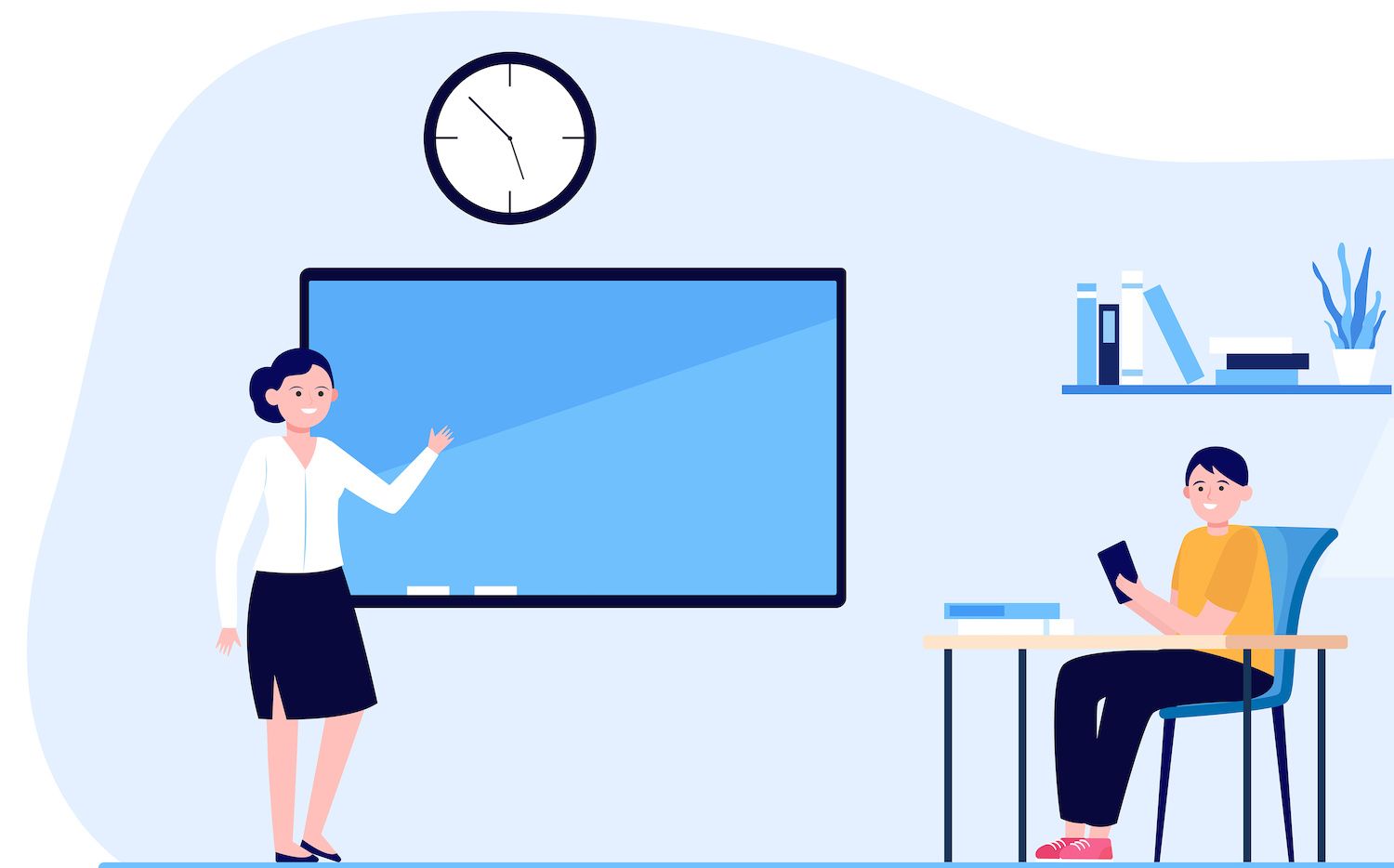
After you've clicked"Send" when you've clicked"Send" following the click of the "Send" button The following information will be sent to you:
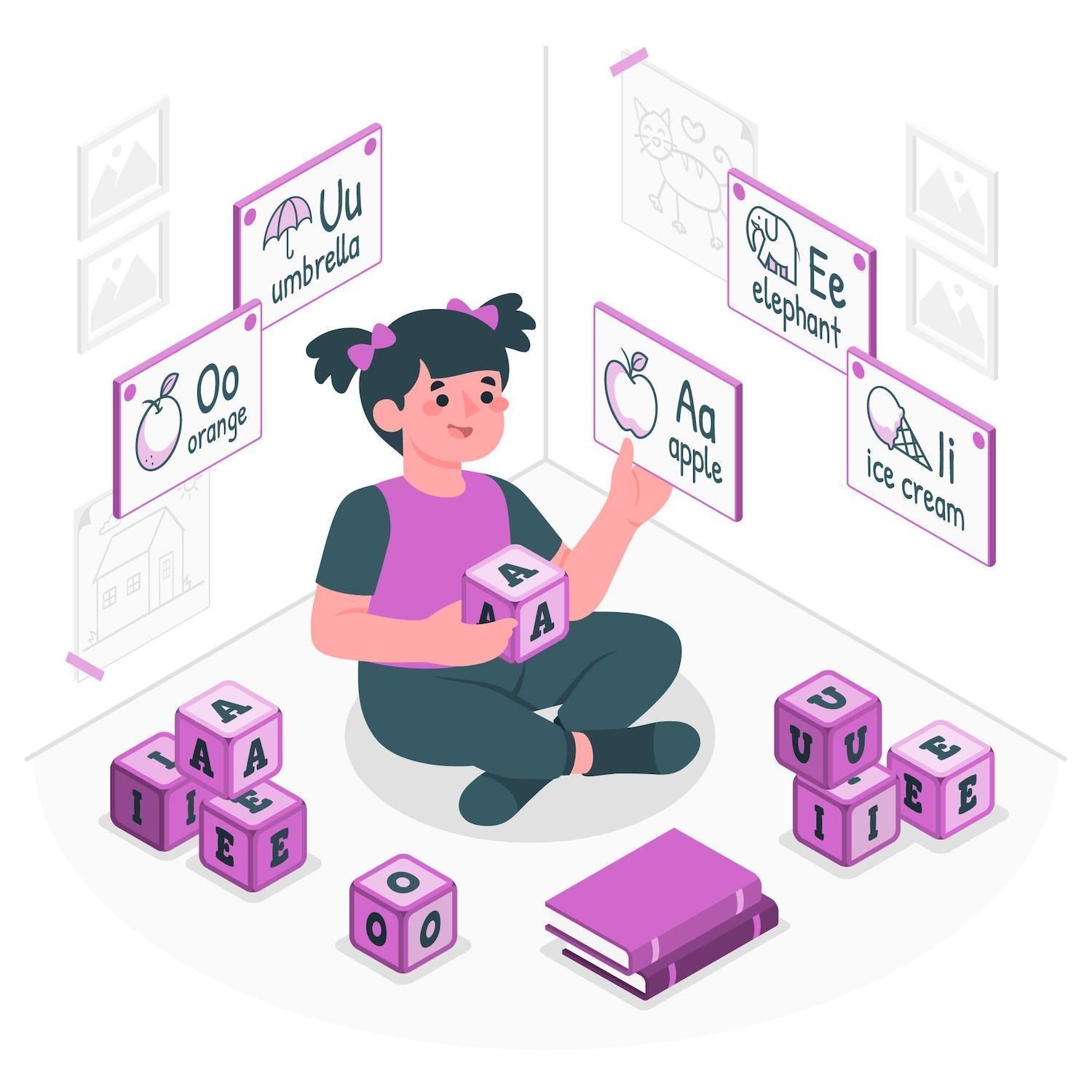
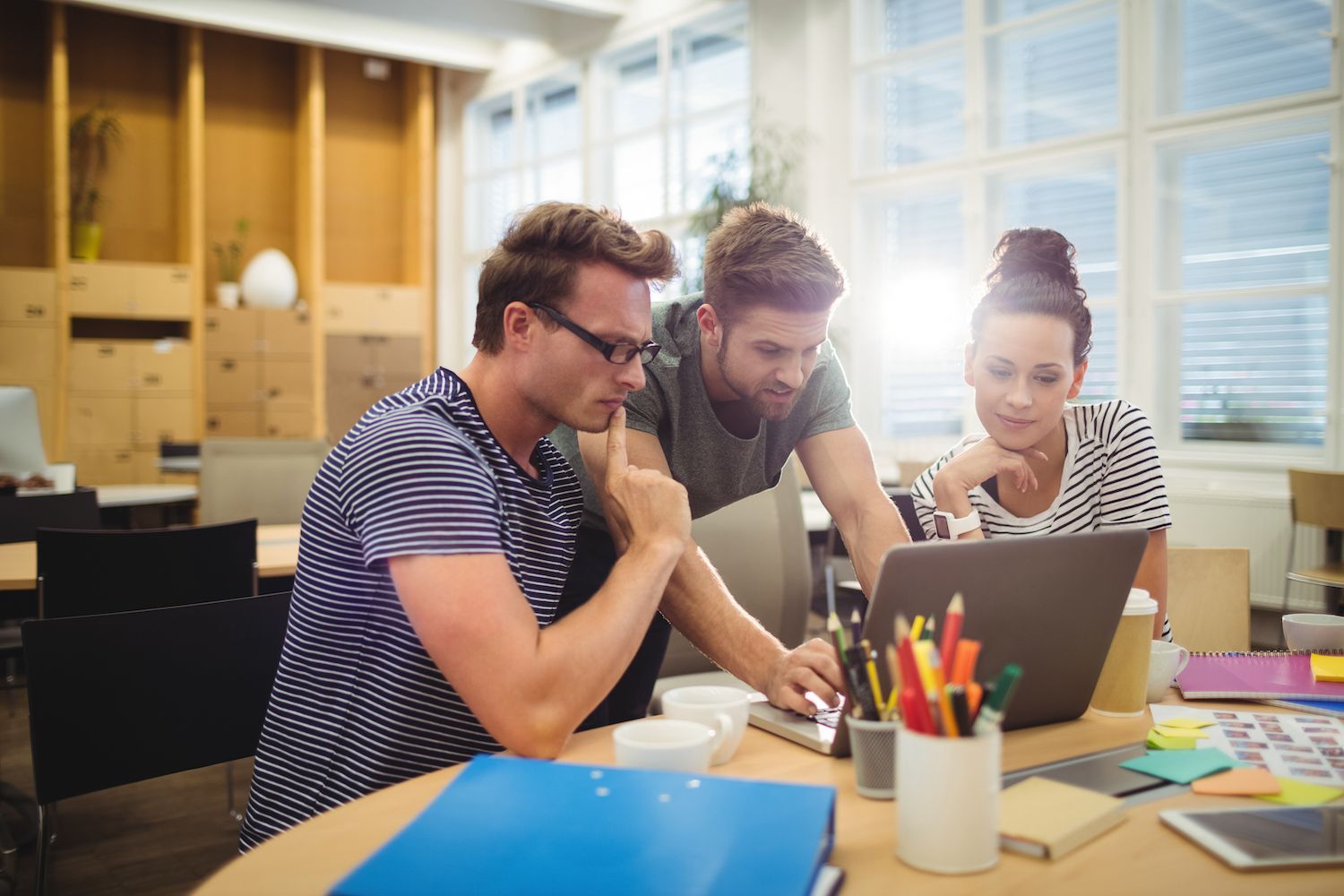
Which is the most efficient method to authenticate APIs with Sanctum?
The security of your API depends on the authenticity. your API. Laravel assists with this by providing security capabilities that form included in the Sanctum token. It's a type of intermediaryware. It encrypts APIs using tokens created when the user logs in to their account with the correct username and password. You must be aware that a user will have access to APIs that is secured with tokens.
The first step in adding authentication is adding the Sanctum package to the above code:
composer require laravel/sanctum
Make sure you have your Sanctum Configuration file
php artisan vendor:publish --provider="Laravel\Sanctum\SanctumServiceProvider"
You can then include the token of Sanctum in middleware. In your app/Http/Kernel.php file, it is recommended to apply these classes and to replace middlewareGroups
by using the following code in order to connect to the middleware group's API.
use Laravel\Sanctum\Http\Middleware\EnsureFrontendRequestsAreStateful;
protected $middlewareGroups = [ 'web' => [ \App\Http\Middleware\EncryptCookies::class, \Illuminate\Cookie\Middleware\AddQueuedCookiesToResponse::class, \Illuminate\Session\Middleware\StartSession::class, // \Illuminate\Session\Middleware\AuthenticateSession::class, \Illuminate\View\Middleware\ShareErrorsFromSession::class, \App\Http\Middleware\VerifyCsrfToken::class, \Illuminate\Routing\Middleware\SubstituteBindings::class, ], 'api' => [ EnsureFrontendRequestsAreStateful::class, 'throttle:api', \Illuminate\Routing\Middleware\SubstituteBindings::class, ], ];
The next step is to develop the UserController. UserController
is later added to an application program to generate an authentication token.
php artisan make:controller UserController
After creating the UserController
, navigate to the app/Http/Controllers/UserController.php file and replace the existing code with the following code:
In order to authenticate it is essential to create an account for an individual user that has seeders. The command generates the tables of users document.
php artisan make:seeder UsersTableSeeder
Inside the database/seeders/UsersTableSeeder.php file, replace the existing code with the following code to seed the user: insert([ 'name' => 'John Doe', 'email' => '[email protected]', 'password' => Hash::make('password') ]);
Now is the time to start implementing the following guidelines:
php artisan db:seed --class=UsersTableSeeder
The final stage of the authentication process is to install the middleware designed to secure the routing. Examine the routes/api.php file, and then add the routing for your item in the. routes/api.php file and include the route that you created from your product in middleware.
use App\Http\Controllers\UserController; Route::group(['middleware' => 'auth:sanctum'], function () Route::apiResource('products', ProductController::class); ); Route::post("login",[UserController::class,'index']);
If you've set up a fresh route with the middleware you'll see an error message coming within the internals of your server whenever you attempt to download the program.
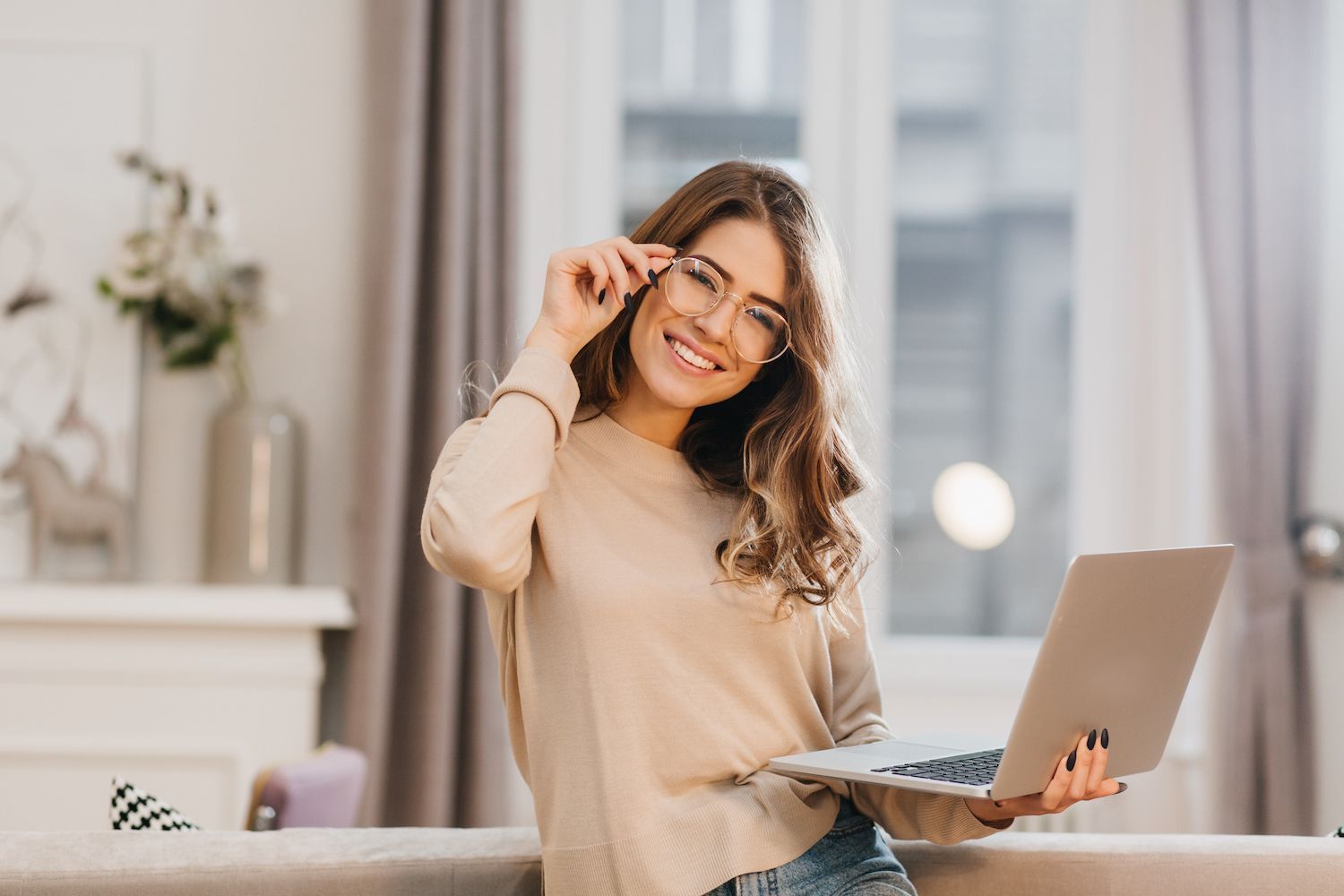
When you've successfully connected with the username you've chosen, you'll be able to receive personal tokens to usage with your account. If you choose to utilize this method, ensure that you include in the HTTP header. It will then verify your identity before working. You can send a POST request to http://127.0.0.1:8000/api/login with the following body:
"email":"[email protected]", "password":"password"
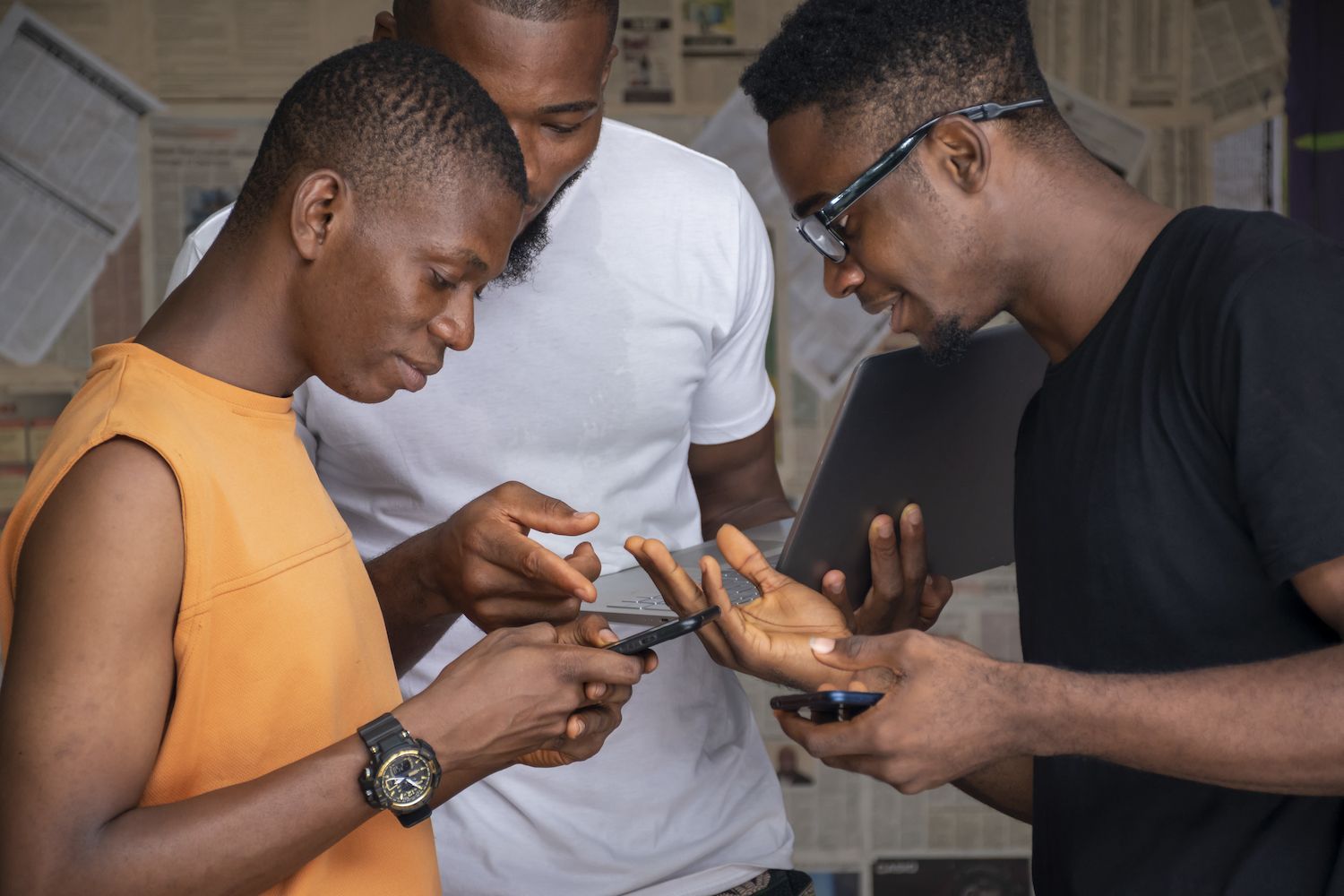
Make use of the token you got as a bearer token, after which you can include it in the header of Authorization.
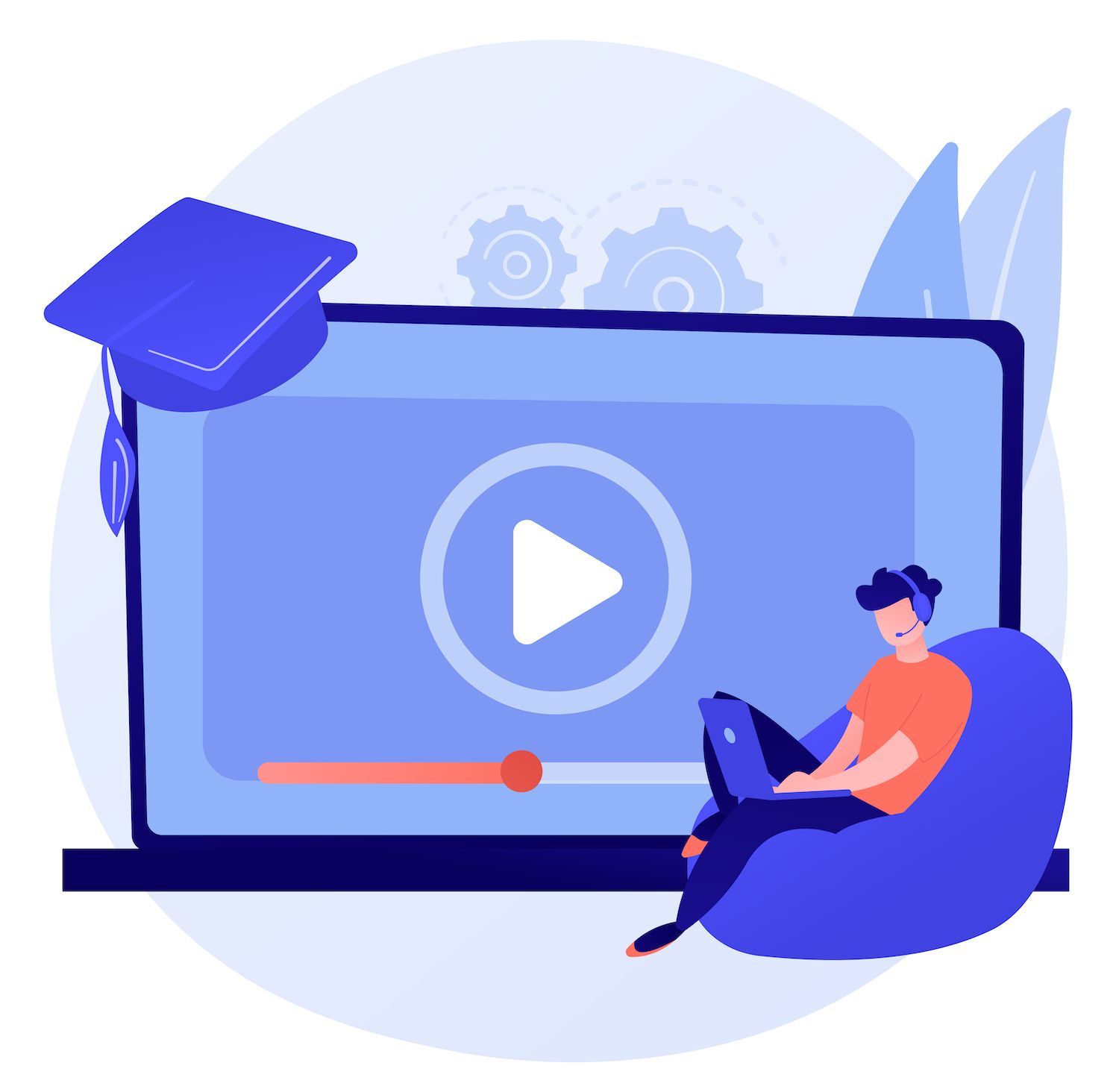
What can you achieve to resolve API Errors?
An error code by itself cannot suffice. A error message that is easily read by anyone is vital. Laravel provides a range of choices to address the issue. Use a fallback, catch block or even an individual response. This code you copy and paste in the UserController UserController
can show the above.
if (!$user || !Hash::check($request->password, $user->password)) return response([ 'message' => ['These credentials do not match our records.'] ], 404);
Summary
The Laravel Eloquent Model makes it simple to create and build the API and then try it out. Mapping objects offers the ability to connect seamlessly to databases.
Additionally, it functions as a middleware. The token Sanctum created by Laravel aids in the protection of APIs within a short period of.
- My dashboard is easy to control and configure inside My dashboard. My dashboard
- Support is accessible 24/7.
- The most secure Google Cloud Platform hardware and network are driven by Kubernetes to enhance performance.
- Premium Cloudflare integration which accelerates the process as well as improve security
- Global reach with more than 35 data centers as plus 227 points of contact around the globe
The article originally appeared on this website
The article first appeared here
This post was first seen here. this site
The post was published on this site.
This post was first seen on here